In this article we are going to see how you can do APIs operations in Flutter with GetX architecture. The topics we will discuss in this article are listed below., so before going to make any delay let’s start…
- What is GetX?
- How to create an app with GetX architecture?
- What is GetConnect?
- How to set up GetConnect?
- How to make REST API requests using GetConnect?
- How to make GraphQL API requests using GetConnect?
- Conclusion
- Resources
What is GetX?
GetX is an open source, extra-light, and powerful solution for developing Flutter apps. State management, intelligent dependency injection, and route management can be accomplished quickly and effectively with it.
GetX is three main basic features which are:
- Performance
- Productivity
- Organisation
Performance
GetX is all about performance and consuming as little resources as possible. It doesn’t use Streams or ChangeNotifiers.
Productivity
GetX has easy syntax, so developers can easily remember those syntaxes. In GetX for any complex problem there are always easier solutions that can save your hours of development and it will provide you maximum app performance.
The developer should generally take care to remove unused resources from volatile memory (RAM). GetX automatically removes unused resources so you don’t have to worry about memory management.
Organization
GetX separates the Views, presentation logic, business logic, dependency injection, and navigation. For navigating between routes, you do not require context, so you do not need a widget tree (visualization).
How to create an app with GetX architecture?
If you don’t know how to create an app using GetX architecture in Flutter, please click on the link below and read my article,
Article: How to create an app with GetX architect in Flutter?
What is GetConnect?
GetConnect makes it easy to make requests and fetch data. In order to communicate with APIs (Application Programming Interface) , you can use GetConnect with the GET/POST/PUT/DELETE/SOCKET methods.
There are already so many packages on pub.dev for API operations such as dio and http, so why GetConnect?
When you are building your app on GetX architecture, you don’t need to install any extra packages like dio & http for APIs operation, GetConnect will take care of everything.
How to set up GetConnect?
Here are some simple steps to set up GetConnect in your Flutter GetX project:
STEP: 1
Create a dependency injection class where we will initialize our GetConnect class.
//Create a file: dependency_injection.dart
import 'package:get/get.dart';
class DependencyInjection {
static void init() async {
Get.put<GetConnect>(GetConnect()); //initializing GetConnect
}
}
STEP: 2
Now, let’s go to main.dart and call DependencyInjection.
void main() {
runApp(const MyApp());
DependencyInjection.init(); //calling DependencyInjection init method
}
To enable GetConnect in our project, we just need to follow these two simple steps.
How to make REST API requests using GetConnect?
For the REST API operations, We wrote a separate class with two methods, one for GET requests and one for POST requests.
//Create a file: rest_api.dart
import 'package:get/get.dart';
class RestAPI{
final GetConnect connect = Get.find<GetConnect>();
//GET request example
Future<dynamic> getDataMethod() async {
Response response = await connect.get('your_get_api_url');
if(response.statusCode == 200) {
return response.body;
}else{
return null;
}
}
//post request example
Future<dynamic> postDataMethod() async {
//body data
FormData formData = FormData({
'field_name': 'field_value',
'field_name': 'field_value',
});
Response response = await connect.post('your_post_api_url', formData);
if(response.statusCode == 200) {
return response.body;
}else{
return null;
}
}
}
Now, in dependencyinjection.dart, we need to call the RestAPI class.
class DependencyInjection {
static void init() async {
Get.put<GetConnect>(GetConnect());
Get.put<RestAPI>(RestAPI()); //initializing REST API class
}
}
Now you can access the RestAPI class anywhere in your code by simply calling:
final RestAPI restAPI = Get.find<RestAPI>();
How to make GraphQL API requests using GetConnect?
First, we need to create a queries.dart file where we will write all our GraphQL query methods.
//Create a file: queries.dart
class GraphqlQuery {
static String languageListQuery(int limit) => '''
{
language {
code
name
native
}
}
''';
static String addLanguageQuery(String code, String name, String native) => '''
mutation {
addLanguageMethod(addLanguage: {
code: $code,
name: $name,
native: $native
}){
status,
message
}
}
}
''';
}
Now, create a separate file for GraphQL API operations,
//Create a file: graph_api.dart
class GraphAPI {
final GetConnect connect = Get.find<GetConnect>();
//GET(query) request example
Future<dynamic> getLanguages() async {
Response response;
try {
response = await connect.query(
GraphqlQuery.languageListQuery(10),
url: "your_api_endpoint",
headers: {'Authorization': 'Bearer paste_your_jwt_token_key_here'},
);
final results = response.body['language'];
if (results != null && results.toString().isBlank == false && response.statusCode == 200) {
return results;
} else {
return null;
}
} catch (e) {
print(e);
}
}
//POST (mutation) request example
Future<dynamic> addLanguage() async {
Response response;
try {
response = await connect.query(
GraphqlQuery.addLanguageQuery('HI', 'Hindi', 'India'),
url: "your_api_endpoint",
headers: {'Authorization': 'Bearer paste_your_jwt_token_key_here'},
);
final results = response.body['addLanguageMethod'];
if (results != null && results.toString().isBlank == false && response.statusCode == 200) {
return results;
} else {
return null;
}
} catch (e) {
print(e);
}
}
}
Now, in dependencyinjection.dart, we need to call the GraphAPI class.
class DependencyInjection {
static void init() async {
Get.put<GetConnect>(GetConnect());
Get.put<RestAPI>(RestAPI());
Get.put<GraphAPI>(GraphPI()); //initializing Graph API class
}
}
Now you can access the GraphAPI class anywhere in your code by simply calling:
final GraphAPI graphAPI = Get.find<GraphAPI>();
Conclusion
Throughout this article, we have seen how to use GetConnect for REST and GraphQL APIs. We don’t require any other dependency, such as http or dio, If you are developing your app in GetX architecture with Flutter.
Resources
Here are some resource links for you to check out.
- https://chornthorn.github.io/getx-docs/
- https://dev.to/this-is-learning/how-to-create-an-app-with-getx-architect-in-flutter-2ehk
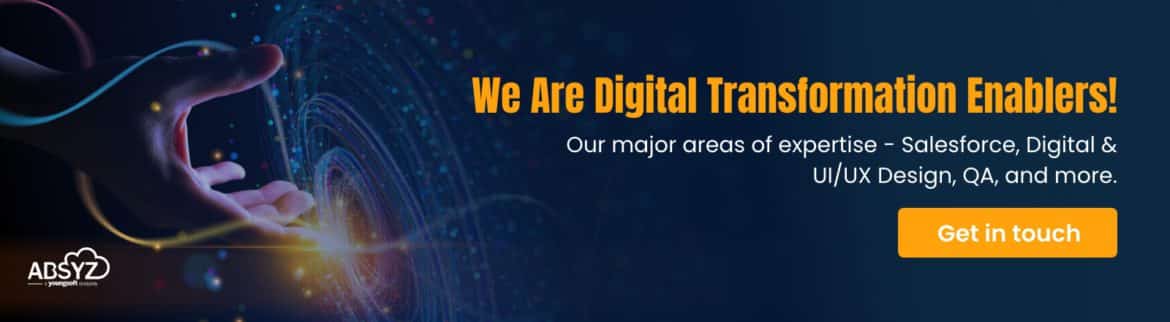