Generally, when we are building a mobile application, we need to store some data locally in the mobile device. At that point, the Hive database comes into play. In this article, we are going to see how you can create a local database in Flutter using the Hive database. So, before we go any further, let’s begin…Â
- What is Hive database?
- Hive database installationÂ
- What are boxes in the hive database?
- Advantages of hive database
- Operations in the hive database
- Conclusion
- Resources
What is Hive Database?
Hive is a lightweight, no-SQL database for flutter and dart applications. Hive is a great choice if you are looking for a straightforward key-value database with few relations. It is also extremely easy to use. It stores data locally on a mobile device. Hive database is inspired by Bitcask.
Hive Database InstallationÂ
Add the following dependencies in the pubspec.yaml:
dependencies:
hive: ^[version]
hive_flutter: ^[version]
What Are Boxes In The Hive Database?
There are boxes for storing all the data in Hive. The box is similar to a table in SQL, but it has no structure and can contain anything.
If the app is small, a single box might be sufficient. If you’re working on a more advanced problem, boxes are a great way to organize your data. You can also encrypt boxes to store sensitive information.
Advantages of Hive Database
- In comparison to SQFlite and SharedPreferences, it is faster in terms of speed and performance.
- Strong encryption built-in.
- Designed to support No-SQL databases
- It does not depend on any native libraries
- Easy-to-use
Operations In The Hive Database
First, we need to initialize the hive database in the main.dart file.
import 'package:flutter/material.dart';
import 'package:hive_database/home_page.dart';
import 'package:hive_flutter/hive_flutter.dart';
void main() async {
await Hive.initFlutter();
await Hive.openBox('hive_local_db');
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Flutter Hive DB',
theme: ThemeData(
// is not restarted.
primarySwatch: Colors.blue,
),
home: HomePage(),
);
}
}
Create user model class (user_model.dart)
class UserModel {
int id;
String username;
String email;
UserModel({
required this.id,
required this.username,
required this.email,
});
Map<String, dynamic> toMap(UserModel userModel) {
Map<String, dynamic> userModelMap = Map();
userModelMap["author"] = userModel.id;
userModelMap["username"] = userModel.username;
userModelMap["email"] = userModel.email;
return userModelMap;
}
factory UserModel.fromMap(Map<String, dynamic> json) => UserModel(
id: json['id'] ?? 0,
username: json['username'] ?? '',
email: json['email'] ?? '',
);
}
Create hive method class (hive_methods.dart)
class HiveMethods {
String hiveBox = 'hive_local_db';
//Adding user model to hive db
addUser(UserModel userModel) async {
var box = await Hive.openBox(hiveBox); //open the hive box before writing
var mapUserData = userModel.toMap(userModel);
await box.add(mapUserData);
Hive.close(); //closing the hive box
}
//Reading all the users data
Future<List<UserModel>> getUserLists() async {
var box = await Hive.openBox(hiveBox);
List<UserModel> users = [];
for (int i = box.length - 1; i >= 0; i--) {
var userMap = box.getAt(i);
users.add(UserModel.fromMap(Map.from(userMap)));
}
return users;
}
//Deleting one data from hive DB
deleteUser(int id) async {
var box = await Hive.openBox(hiveBox);
await box.deleteAt(id);
}
//Deleting whole data from Hive
deleteAllUsers() async {
var box = await Hive.openBox(hiveBox);
await box.clear();
}
}
Now create the home page screen (home_page.dart)
import 'package:flutter/material.dart';
import 'package:hive_database/hive_methods.dart';
import 'package:hive_database/user_model.dart';
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
final HiveMethods hiveMethods = HiveMethods();
List<UserModel> users = [];
bool isLoading = true;
@override
void initState() {
super.initState();
fetchUsers();
}
void fetchUsers() async {
var usersData = await hiveMethods.getUserLists();
if (usersData.isNotEmpty) {
users.addAll(usersData);
}
setState(() {
isLoading = false;
});
}
void addUser() {
hiveMethods.addUser(
UserModel(id: 1, username: 'Sarfaraj', email: 'sarfaraj@gmail.com'));
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Hive DB'),
),
body: isLoading
? const Center(child: CircularProgressIndicator())
: SingleChildScrollView(
child: ListView.builder(
shrinkWrap: true,
physics: const ClampingScrollPhysics(),
itemCount: users.length,
itemBuilder: (BuildContext context, index) {
return Card(
child: ExpansionTile(
expandedAlignment: Alignment.centerLeft,
expandedCrossAxisAlignment: CrossAxisAlignment.start,
backgroundColor: Colors.white,
childrenPadding:
const EdgeInsets.fromLTRB(15, 0, 15, 10),
title: Text(users[index].username),
children: [
Text('ID: ${users[0].id}'),
Text('Username: ${users[0].username}'),
Text('Email: ${users[0].email}')
],
),
);
},
),
),
floatingActionButton: FloatingActionButton(
onPressed: () {
addUser();
},
tooltip: 'Add User',
child: const Icon(Icons.add),
));
}
}
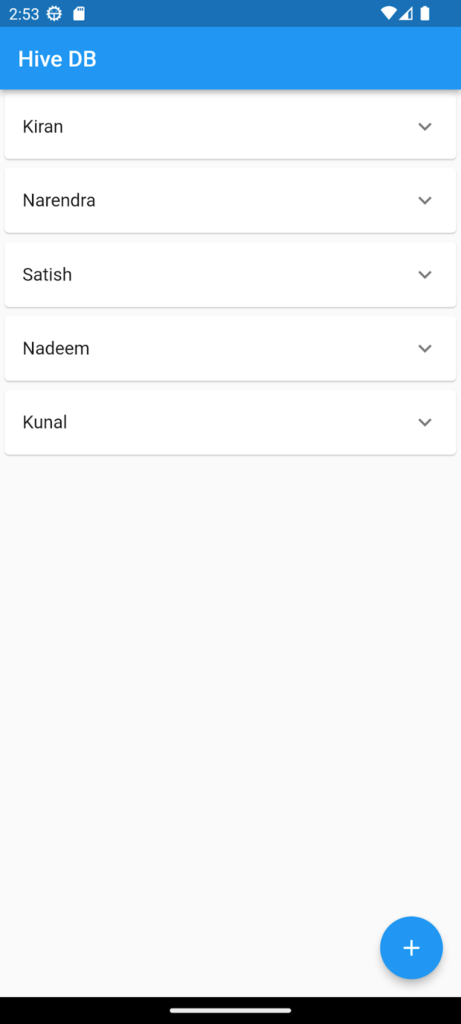
Here is the link to the github repo where you can download the code,
GitHub: https://github.com/sarfaraj-absyz/hive_database_operation.git
Conclusion
We saw how to integrate a Hive database in Flutter throughout this article. And also, we saw how simple, fast, and secure it is to integrate a Hive database into a Flutter application to store data locally on mobile. We hope you found this article useful in setting up a hive database in your next Flutter project.
Resources
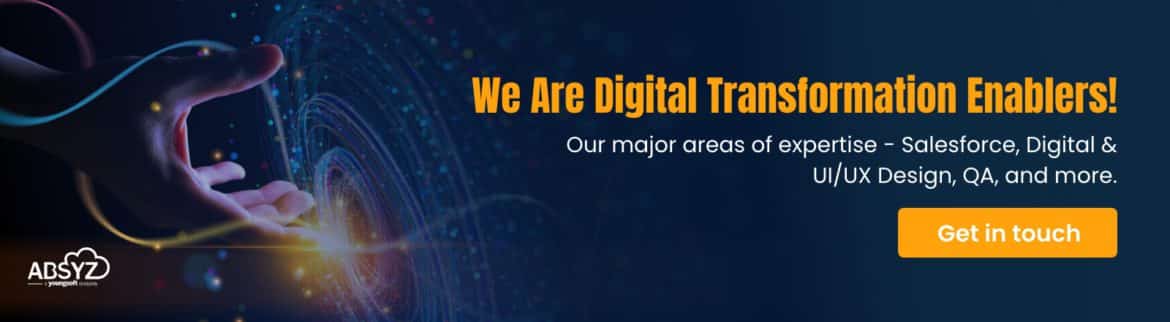