In this blog, I am going to take you through the process of how to schedule a report from apex class. While I was working on my last project I came across a scenario where I need to schedule a report from the apex. This blog takes you through the simplest way possible to achieve this requirement.
Using analytics REST API we can do the following things :
- Create, update and retrieve the Salesforce Report and Dashboard.
- Modify and schedule trends in Analytics report snapshot.
- Retrieve a list of dataset versions.
- Retrieve a list of dependencies for an application.
In order to schedule a report first, we need to understand ‘reports’ namespace.
Reports namespace contain a number of classes through which we can avail the same level of data access as in case of using Salesforce Reports and DashBoards REST API.
There are two ways of running a report through apex.
- Synchronously
- Asynchronously
Synchronous Way of running  reports:
If you want to run reports synchronously we have to use ReportManager class. In this, we need to use runreport() Method. The Return type of this report must be ReportResult class. This class contains results after running a report.
Check the below code for running report synchronously.
[sourcecode language=”java”]
public class ScheduleReport {public ScheduleReport(){
list reportList=[select id, name from report where name=’DemoReport’’];
// To get the report Id.
string reportId=(string) reportList.get(0).get(‘Id’);
//To Run the report.
reports.ReportResults reportResult=reports.ReportManager.runReport(reportId, true);
system.debug(‘reportResult—>’+reportResult);
}
}
[/sourcecode]
Asynchronously way of running reports:
If you want to run reports asynchronously we have to use ReportManager class similar to Synchronous way of running reports. Here you need to use runAsyncReport() method instead of runReport() method.
Advantages of running reports Asynchronously:
- We can lower the risk of reaching the timeout limit when we run reports.
- Salesforce API timeout limit does not apply for asynchronous runs.
- Salesforce Reports and dashboards API can handle higher number of asynchronous run requests at a time.
Check the below code to Schedule a report Asynchronously
[sourcecode language=”java”]
public class ScheduleReport {public ScheduleReport(){
list reportList=[select id, name from report where name=’DemoReport’’];
string reportId=(string) reportList.get(0).get(‘Id’);
Reports.ReportInstance instance = Reports.ReportManager.runAsyncReport(reportId, true);
System.debug(‘Asynchronous instance: ‘ + instance);
}
}
[/sourcecode]
Now we need to write a schedulable class to schedule our report.
Below is the code for schedule class.
[sourcecode language=”java”]
global class ScheduleReportClass implements Schedulable{global void Execute(SchedulableContext context){
ScheduleReport sch=new ScheduleReport();
}
}
[/sourcecode]
Let us consider a scenario where I need to schedule a report every weekday at 1PM.
Below is the code for scheduling
[sourcecode language=”java”]
ScheduleReportClass sch=new ScheduleReportClass();
string cronExp=’0 0 13 ? * MON-FRI’;
system.schedule(‘schedule report’, cronExp, sch);[/sourcecode]
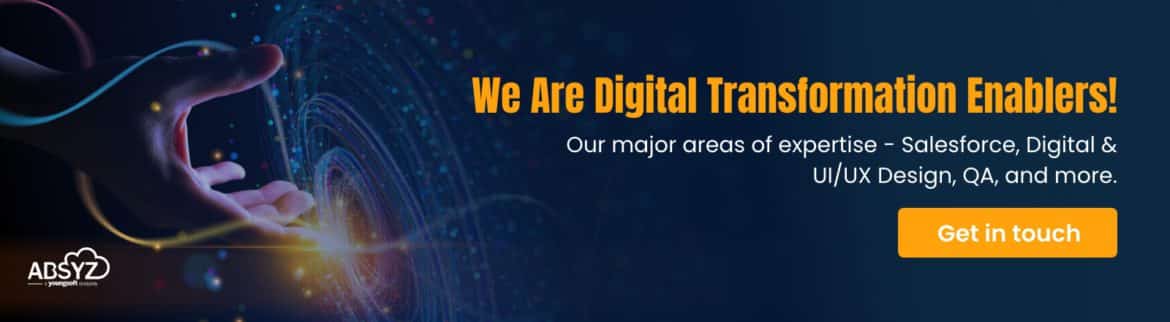