Calling controller from LWC:
Lightning web components can import methods from Apex classes into the JavaScript classes using ES6 import.Once after importing the apex class method you can able to call the apex methods as functions into the component by calling either via the wire service or imperatively.
Import Syntax
import apexMethod from’@salesforce/apex/Namespace.Classname.apexMethod’;
apexMethod : This identifies the Apex method name.
Classname : The Apex class name.
Namespace : The namespace of the Salesforce organization
To expose an Apex method to a Lightning web component, the method must be static and either global or public. Annotate the method with @AuraEnabled
[sourcecode language=”java”]
public with sharing class ContactAuraService {
    public ContactAuraService() {
    }
    @AuraEnabled(cacheable=true)
    public static List<sObject> getContactList(){
        String query = ‘select id,Name,Email,Phone From Contact’;
        return database.query(query);
    }
}
[/sourcecode]
After importing the apex class method you can able to call the apex methods as functions into the component by calling either via the wire service or imperatively. We have three ways to call Apex method
1.Wire a property
2.Wire a function
3.Call a method imperatively.
1.Wire a property:
<!–LWCWireExample.html–>
[sourcecode language=”java”]
<template>
    <lightning-card title=”Wire a property” icon-name=”standard:contact”>
            <div class=”slds-m-around_medium”>
                <template if:true={contacts.data}>
                    <template for:each={contacts.data} for:item=”con”>
                        <p key={con.Id}>{con.Name}</p>
                    </template>
                </template>
            </div>
        </lightning-card>
</template>
[/sourcecode]
<!–LWCWireExample.js–>
[sourcecode language=”java”]
import { LightningElement, wire } from ‘lwc’;
import getContactList from ‘@salesforce/apex/ContactAuraService.getContactList’;
Â
export default class LWCWireExample extends LightningElement {
    @wire(getContactList) contacts;
}
[/sourcecode]
<!–LWCWireExample.js-meta.xml–>
[sourcecode language=”java”]
<?xml version=”1.0″ encoding=”UTF-8″?>
<LightningComponentBundle xmlns=”http://soap.sforce.com/2006/04/metadata” fqn=”LWCWireExample”>
    <apiVersion>45.0</apiVersion>
    <isExposed>true</isExposed>
    <masterLabel>Wire a property For Contact List</masterLabel>
     <targets>
    <target>lightning__AppPage</target>
    <target>lightning__RecordPage</target>
  </targets>
</LightningComponentBundle>
[/sourcecode]
2.Wire a function:
This mechanism is needed if you want to pre-process the data before going to the Lightning App.
<!–LWCWireExample.js–>
[sourcecode language=”java”]
import { LightningElement, wire,track } from ‘lwc’;
import getContactList from ‘@salesforce/apex/ContactAuraService.getContactList’;
export default class LWCWireExample extends LightningElement {
    @track contacts;
    @track error;
    @wire(getContactList)
    wiredContacts({ error, data }) {
        if (data) {
            this.contacts = data;
        } else if (error) {
            this.error = error;
        }
    }
}
[/sourcecode]
<!–LWCWireExample.html–>
[sourcecode language=”java”]
<template>
    <lightning-card title=”Wire To a function Example” icon-name=”standard:contact”>
         <div class=”slds-m-around_medium”>
            <template if:true={contacts}>
                <template for:each={contacts} for:item=”con”>
                    <p key={con.Id}> {con.Name} </p>
                </template>
            </template>
            <template if:true={error}>
                {error}
            </template>               Â
        </div>
    </lightning-card>
</template>
[/sourcecode]
<!–LWCWireExample.js-meta.xml→
[sourcecode language=”java”]
<?xml version=”1.0″ encoding=”UTF-8″?>
<LightningComponentBundle xmlns=”http://soap.sforce.com/2006/04/metadata” fqn=”LWCWireExample”>
    <apiVersion>45.0</apiVersion>
    <isExposed>true</isExposed>
    <masterLabel>Wire to a function For Contact List</masterLabel>
     <targets>
    <target>lightning__AppPage</target>
    <target>lightning__RecordPage</target>
  </targets>
</LightningComponentBundle>
[/sourcecode]
3.Call a method imperatively:
If an Apex method is not annotated with cacheable=true, you must call it imperatively.
<!–LWCWireExample.js→
[sourcecode language=”java”]
import { LightningElement, wire,track } from ‘lwc’;
import getContactList from ‘@salesforce/apex/ContactAuraService.getContactList’;
export default class LWCWireExample extends LightningElement {
    @track contacts;
    @track error;
handleLoad() {
getContactList()
.then(result => {Â
this.contacts = result;
this.error = undefined;
})
.catch(error => {
this.error = error;
            this.contacts = undefined;
});
}
}
[/sourcecode]
<!–LWCWireExample.html–>
[sourcecode language=”java”]
<template>
     <lightning-card title=”Call a method imperatively Example” icon-name=”standard:contact”>
         <div class=”slds-m-around_medium”>
<p class=”slds-m-bottom_small”>
                <lightning-button label=”Get Contacts” onclick={handleLoad}></lightning-button>
            </p>
            <template if:true={contacts}>
                <template for:each={contacts} for:item=”con”>
                    <p key={con.Id}> {con.Name} </p>
                </template>
            </template>
            <template if:true={error}>
                {error}
            </template>               Â
        </div>
    </lightning-card>
</template>
[/sourcecode]
<!–LWCWireExample.js-meta.xml–>
[sourcecode language=”java”]
<?xml version=”1.0″ encoding=”UTF-8″?>
<LightningComponentBundle xmlns=”http://soap.sforce.com/2006/04/metadata” fqn=”LWCWireExample”>
    <apiVersion>45.0</apiVersion>
    <isExposed>true</isExposed>
    <masterLabel>Call a method imperatively</masterLabel>
     <targets>
    <target>lightning__AppPage</target>
    <target>lightning__RecordPage</target>
  </targets>
</LightningComponentBundle>[/sourcecode]
Lightning Web Components with Aura Components:
In an Aura component, to refer to either Aura components or Lightning web components, use camel case with a colon separating the namespace and the component name.Aura components can contain Lightning web components.However, the opposite doesn’t apply.Lightning web components can’t contain Aura components.
<!–ContactList.cmp→
[sourcecode language=”java”]
<aura:component>
    <lightning:card title=”Aura component” iconName=”custom:custom14″ />
    <c:lWCWireExample/>
</aura:component>
[/sourcecode]
<!–TestApp.app–>
[sourcecode language=”java”]
<aura:application extends=”force:slds”>
    <c:ContactList/>
</aura:application>[/sourcecode]
LDS in Lightning Web Component:
LDS is the Lightning Components counterpart to the Visualforce standard controller, providing access to the data displayed on a page. For Lightning components, Salesforce added a new Feature – Lightning Data Service (LDS) in its Winter ’17 release. We can use LDS in our Lightning components to perform CRUD operations like create,read,update a record without use of any Apex code.Now we see how to implement LDS in LWC.
Create Record through LDS :
First import the createRecord method from ‘lightning/uiRecordApi’ in client side controller.
Syntax:
import { createRecord } from ‘lightning/uiRecordApi’;
<!–accountMangerLds.html–>
[sourcecode language=”java”]
<template>Â Â Â
 <lightning-card title=”Account Manager”>
        <lightning-layout>
                <lightning-layout-item size=”6″ padding=”around-small”>
<lightning-card title=”Create Account”>
<lightning-input label=”Id” disabled value={accountId}></lightning-input>
<lightning-input name=”Account Name”  label=”Account Name” type=”text” onchange= {accountNameHandler}></lightning-input>
<lightning-input name=”Account Phone” Â label=”Account Phone” type=”phone” onchange= {accountPhoneHandler}></lightning-input>
<lightning-button label=”Create Account” variant=”brand” onclick= {createAccount}></lightning-button>
                       </lightning-card>
                 </lightning-layout-item>
          <lightning-layout-item size=”6″ padding=”around-small”>
     </lightning-layout-item>
   </lightning-layout>
</lightning-card>
</template>
[/sourcecode]
<!–accountMangerLds.js–>
[sourcecode language=”java”]
import { LightningElement, api } from ‘lwc’;
import { createRecord } from ‘lightning/uiRecordApi’;
export default class AccountManagerLDS extends LightningElement {
    @api accountName;
    @api accountPhone;
    @api accountId;
    accountNameHandler(event){
        this.accountId = undefined;
        this.accountName = event.target.value;
    }
    accountPhoneHandler(event){
        this.accountId = undefined;
        this.accountPhone = event.target.value;
    }
    createAccount(){
        const fields = {‘Name’ : this.accountName,’Phone’ : this.accountPhone};
        const recordInput = {apiName:’Account’,fields};
        createRecord(recordInput)
           .then(response=>{
            this.accountId = response.id;
           })
           .catch(()=>{
          Â
           });
    }
}[/sourcecode]
<!–accountMangerLds.js-meta.xm–>
[sourcecode language=”java”]
<?xml version=”1.0″ encoding=”UTF-8″?>
<LightningComponentBundle xmlns=”http://soap.sforce.com/2006/04/metadata” fqn=”accountManagerLDS”>
    <apiVersion>45.0</apiVersion>
    <isExposed>true</isExposed>
    <masterLabel>Create Record LDS</masterLabel>
     <targets>
    <target>lightning__AppPage</target>
    <target>lightning__RecordPage</target>
  </targets>
</LightningComponentBundle>[/sourcecode]
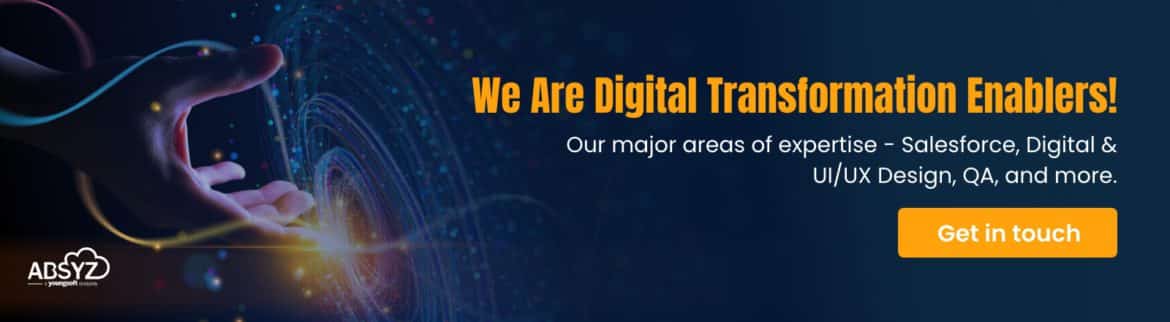