Here we are going to send the meeting invites from salesforce using ICS calendar to our outlook. In general ICS is the iCalendar/.ics/.ical file and it is used to store the calendar information. Whenever you export anything a outlook, google, etc., calendar it will automatically save as .ics file.
New and updated meetings are sent to participants using an email notification from particular person. This requires diligent effort from all participants to update their local calendars manually. The idea is to automatically generate an ICS file with the meeting details and attach it to the notification email whenever an meeting is scheduled or rescheduled. This would allow participants to see the appointment into their calendars.
Let’s assume we have Meeting object in our Org with the following fields
- Subject – text field
- Meeting Leader – email field
- Objective of meeting – picklist field
- start – Date/Time field
- End – Date/Time field
Now we are trying to send the meeting invite after inserting or updating the record in salesforce. For that i was using the trigger whenever the record in inserted or updated.
Check the below code to send the ICS calendar invite..!!
[sourcecode language="java"] trigger meeting on Meeting__c (after Insert,after Update) { list<Meeting__c> meetList= new list<Meeting__c>(); for(Meeting__c meet:trigger.new){ meetList.add(meet); } AP_Meeting.sendInvite(meetList); } [/sourcecode]
Below is the helper class..!!
[sourcecode language="java"] public class AP_Meeting{ public static void sendInvite(list<Meeting__c> meetingList) { list<string> UserEmailList = new list<string> (); string title; string meetingTitle; for(Meeting__c meet:meetingList){ UserEmailList.add(meet.MeetingLeader__c); meetingTitle=meet.name+': '+meet.Subject__c; title=meet.Subject__c; Messaging.SingleEmailMessage mail = new Messaging.SingleEmailMessage(); mail.setToAddresses(UserEmailList); mail.setPlainTextBody('This to inform that we have meeting related to particular problem'); mail.setSubject(title); Messaging.EmailFileAttachment attach = new Messaging.EmailFileAttachment(); attach.filename = 'meeting.ics'; attach.ContentType = 'text/calendar; charset=utf-8; method=REQUEST'; //PUBLISH';// attach.inline = true; /***************************/ attach.body = invite(UserEmailList, meet.MeetingLeader__c, 'Harish Lingam', meet.Subject__c, meet.start__c, meet.end__c, meet.createdDate, system.now(), meet.ObjectiveOfMeeting__c, meet.LastModifiedDate); mail.setFileAttachments(new Messaging.EmailFileAttachment[] { attach }); Messaging.SendEmailResult[] er = Messaging.sendEmail(new Messaging.Email[] { mail }); } } public static Blob invite(List<String> emailsList, String Organiser, string name, string subject, dateTime startDate, dateTime endDate, dateTime createdDate, string description, dateTime lastmodifiedDat) { String txtInvite = ''; string startdateTime; string enddateTIme; string createdDateTime; string lastmodifiedDatTime; startdateTime = startDate.formatGMT('yyyyMMdd'T'HHmmss'Z''); enddateTIme = endDate.formatGMT('yyyyMMdd'T'HHmmss'Z''); createdDateTime = createdDate.formatGMT('yyyyMMdd'T'hhmmss'Z''); lastmodifiedDatTime = lastmodifiedDat.formatGMT('yyyyMMdd'T'hhmmss'Z''); txtInvite += 'BEGIN:VCALENDARn'; txtInvite += 'PRODID:-//Microsoft Corporation//Outlook 16.0 MIMEDIR//ENn'; txtInvite += 'VERSION:2.0n'; txtInvite += 'CALSCALE:GREGORIANn'; txtInvite += 'METHOD:REQUESTn'; txtInvite += 'REPLAY:ACCEPTEDn'; txtInvite += 'BEGIN:VEVENTn'; txtInvite += 'ATTENDEEn'; txtInvite += 'CN=' + subject + 'n'; for (String email: emailsList) { txtInvite += 'ATTENDEE:' + email + 'n'; } txtInvite += 'X-MS-OLK-FORCEINSPECTOROPEN:TRUEn'; txtInvite += 'X-WR-RELCALID:{0000002E-9CDF-9CE8-AD4C-66FC0A5A25F7}n'; txtInvite += 'CLASS:PUBLICn'; txtInvite += 'CREATED:' + createdDateTime+'n'; txtInvite += 'DTEND:' + enddateTIme+'n'; txtInvite += 'DTSTART:' + startdateTime+'n'; txtInvite += 'LAST-MODIFIED:' + lastmodifiedDatTime+'n'; txtInvite += 'ORGANIZER;CN=' + name + ':mailto:' + Organiser + 'n'; txtInvite += 'RSVP=TRUEn'; txtInvite += 'ROLE=REQ-PARTICIPANTn'; txtInvite += 'PARTSTAT=NEEDS-ACTIONn'; txtInvite += 'CN=' + subject + ':mailto:' + Organiser + 'n'; txtInvite += 'LOCATION:Skypen'; txtInvite += 'PRIORITY:5n'; txtInvite += 'SEQUENCE:0n'; txtInvite += 'SUMMARY:n'; txtInvite += 'STATUS:NEEDS-ACTIONn'; txtInvite += 'LANGUAGE=en-us:n'; txtInvite += 'TRANSP:OPAQUEn'; txtInvite += 'UID:4036587160834EA4AE7848CBD028D1D200000000000000000000000000000000n'; txtInvite += 'X-ALT-DESC;FMTTYPE=text/html:<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 3.2//EN"><HTML><HEAD><META NAME="Generator" CONTENT="MS Exchange Server version 08.00.0681.000"><TITLE></TITLE></HEAD><BODY><!-- Converted from text/plain format --></BODY></HTML>n'; txtInvite += 'X-MICROSOFT-CDO-BUSYSTATUS:BUSYn'; txtInvite += 'X-MICROSOFT-CDO-IMPORTANCE:1n'; txtInvite += 'BEGIN:VALARMn'; txtInvite += 'TRIGGER:-PT15Mn'; txtInvite += 'ACTION:DISPLAYn'; txtInvite += 'STATUS:CONFIRMEDn'; txtInvite += 'DESCRIPTION:Remindern'; txtInvite += 'END:VALARMn'; txtInvite += 'END:VEVENTn'; txtInvite += 'END:VCALENDAR'; return Blob.valueOf(txtInvite); } } [/sourcecode]
Now lets create a record in the org. fill the information needed…!!
Now it will directly create a meeting calendar invite in outlook with the details provided. Make sure the all the details filled whatever it is we have used in the code.
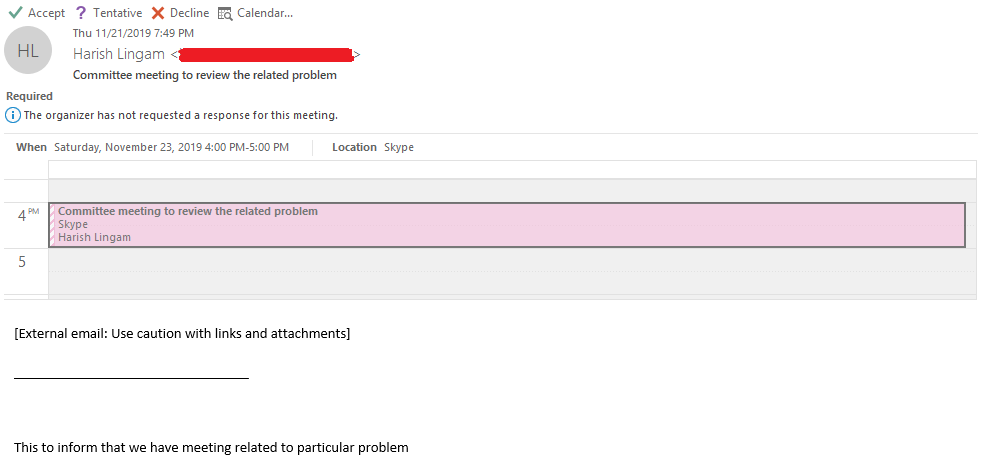
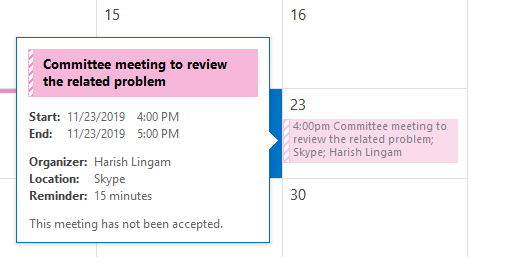
Now we can check calendar for invite.
Now if you want to reschedule the meeting, for that we need to update the record, by updating Start and End time. Now we have rescheduled the meeting to the next day and now the calendar is updated,
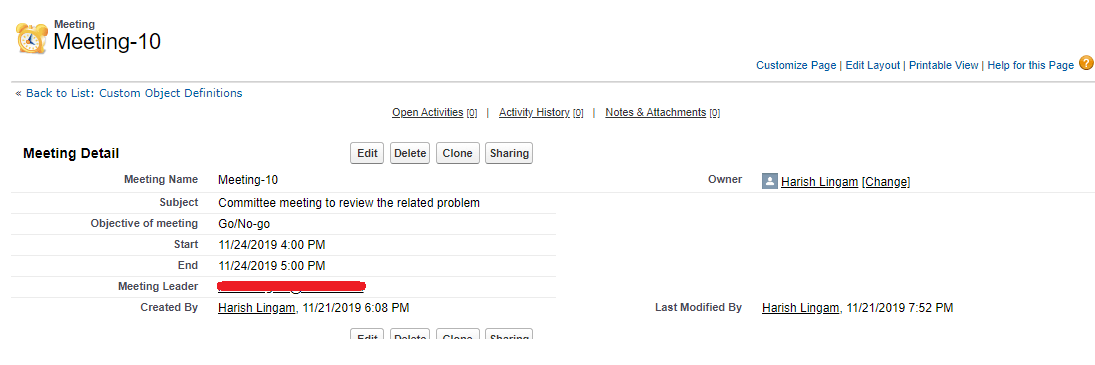
So now you will receive new email with the updated calendar info. Now check the calendar for the updated information.
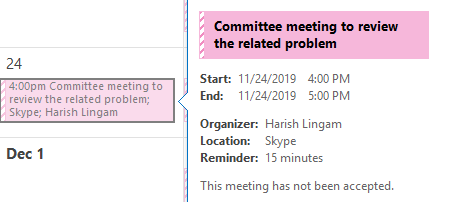
Finally you can add the email template in what ever format you need it, you can also send to the bunch of the people by adding them to the public groups.
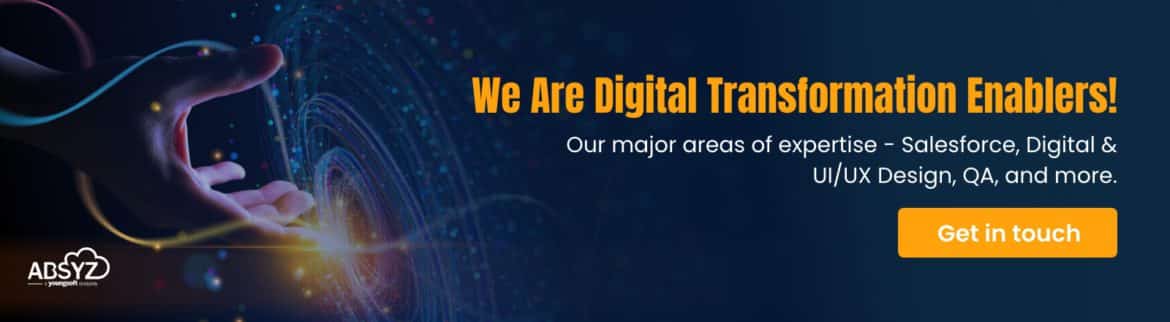