In this blog, we will be learning to use Modal/ Popup in Lightning Web Component. We will be using SLDS classes that helps in showing or hiding the Modal.
The below classes help in showing and hiding of the modal
- slds-fade-in-open – Shows the modal
- slds-backdrop_open – Shows the backdrop(greyed out)
So, adding and removing these classes at an action will show/ hide the modal accordingly.
In this example, we will be making a Modal component and show the data required by checking the sObject of the current record page it is being used. So, this component can be used at any sObject record page, and the required server call can be performed.
Let’s have a look at the components.
Apex Class
Modal_LWC_controller.cls
This class checks the sObject type and calls the function required for that sObject
/**
* @File Name : Modal_LWC_controller.cls
* @Description :
* @Author : Abhishek Kumar
* @Group :
* @Last Modified By : abhishek.kumar@absyz.com
* @Modification Log :
* Ver Date Author Modification
* 1.0 19/10/2019 Abhishek Kumar 1.0
**/
public with sharing class Modal_LWC_controller {
@AuraEnabled(cacheable=true)
public static WrapperClass fetchWrapperData(Id sObjectId){
WrapperClass wrapperClassVar = new WrapperClass();
if(sObjectId.getSobjectType() == Schema.Account.SObjectType){
wrapperClassVar.contactList = Modal_LWC_controller.getRelatedContacts(sObjectId);
}else if(sObjectId.getSobjectType() == Schema.Contact.SObjectType){
wrapperClassVar.accId = Modal_LWC_controller.getAccId(sObjectId);
}
System.debug('wrapperClassVar-->>'+wrapperClassVar);
return wrapperClassVar;
}
public static List<Contact> getRelatedContacts(String accId){
return [SELECT Id, Name, Phone, Email FROM Contact WHERE AccountId =:accId];
}
public static String getAccId(String contactId) {
return [SELECT Id, AccountId FROM Contact WHERE Id=:contactId].AccountId;
}
public class WrapperClass {
@AuraEnabled
public List<Contact> contactList {get; set;}
@AuraEnabled
public Id accId{get; set;}
public wrapperClass() {
this.contactList = new List<Contact>();
}
}
}
LWC Components
modal_LWC.html
<!--
@File Name : modal_LWC.html
@Description :
@Author : Abhishek Kumar
@Group :
@Last Modified By : abhishek.kumar@absyz.com
@Modification Log :
Ver Date Author Modification
1.0 19/10/2019 Abhishek Kumar 1.0
-->
<template>
<lightning-button variant="brand" label={btnLabel} title={btnLabel} onclick={openModal} class="slds-m-left_x-small"></lightning-button>
<section role="dialog" tabindex="-1" aria-labelledby="modal-heading-01" aria-modal="true" aria-describedby="modal-content-id-1" class={modalClass}>
<div class="slds-modal__container">
<header class="slds-modal__header">
<h2 id="modal-heading-01" class="slds-modal__title slds-hyphenate">{modalHeader}</h2>
</header>
<div class="slds-modal__content slds-p-around_medium" id="modal-content-id-1">
<template if:true={isContact}>
<lightning-record-view-form
record-id={accountId}
object-api-name="Account">
<div class="slds-grid">
<div class="slds-col slds-size_1-of-2">
<lightning-output-field field-name="Name"></lightning-output-field>
<lightning-output-field field-name="Website"></lightning-output-field>
</div>
<div class="slds-col slds-size_1-of-2">
<lightning-output-field field-name="Potential_Value__c"></lightning-output-field>
<lightning-output-field field-name="Most_Recent_Closed_Date__c"></lightning-output-field>
</div>
</div>
</lightning-record-view-form>
</template>
<template if:false={isContact}>
<div class="slds-border_left slds-border_right slds-border_top">
<lightning-datatable
key-field="id"
data={contactList}
show-row-number-column
row-number-offset={rowOffset}
hide-checkbox-column
resize-column-disabled
columns={columns}>
</lightning-datatable>
</div>
</template>
</div>
<footer class="slds-modal__footer">
<button class="slds-button slds-button_neutral" onclick={closeModal}>Cancel</button>
<button class="slds-button slds-button_brand" disabled>Save</button>
</footer>
</div>
</section>
<div class={modalBackdropClass}></div>
</template>
modal_LWC.js
import { LightningElement, track, api } from 'lwc';
import { ShowToastEvent } from 'lightning/platformShowToastEvent'
import fetchWrapperData from '@salesforce/apex/Modal_LWC_controller.fetchWrapperData';
const columns = [
{ label: 'Name', fieldName: 'Name' },
{ label: 'Email', fieldName: 'Email', type: 'email' },
{ label: 'Phone', fieldName: 'Phone', type: 'phone' }
];
export default class Modal_LWC extends LightningElement {
@track columns = columns;
@track accountId;
@track contactList;
@track rowOffset = 0;
@track error;
@track btnLabel = '';
@track modalHeader = '';
@track isContact = false;
@track modalClass = 'slds-modal ';
@track modalBackdropClass = 'slds-backdrop ';
@api recordId; //Stores recordId
@api objectApiName; //Stores the current object API Name
connectedCallback() { //doInit() function, Dynamically changing the modal header and button label as per sObject, and also assigning the isContact property as per sObject
if(this.objectApiName === 'Account'){
this.isContact = false;
this.modalHeader = 'Related Contacts';
this.btnLabel = 'See Related Contacts';
}else if(this.objectApiName === 'Contact'){
this.isContact = true;
this.modalHeader = 'Account Details';
this.btnLabel = 'See Account Details';
}
}
//Adds the classes that shows the Modal and does server calls to show the required data
openModal() {
this.modalClass = 'slds-modal slds-fade-in-open';
this.modalBackdropClass = 'slds-backdrop slds-backdrop_open';
fetchWrapperData({sObjectId: this.recordId})
.then(result => { //Returns the wrapper
if(result.accId != null){
this.accountId = result.accId;
this.isContact = true;
}else{
this.contactList = result.contactList;
this.isContact = false;
}
})
.catch(error => {
const event = new ShowToastEvent({
title: 'Error Occured',
message: 'Error: '+error.body.message,
variant: 'error'
});
this.dispatchEvent(event); //To show an error Taost if error occurred while the APEX call
});
}
//Removes the classes that hides the Modal
closeModal() {
this.modalClass = 'slds-modal ';
this.modalBackdropClass = 'slds-backdrop ';
}
}
Alternatively, we can use <template if:false> for showing/ hiding the modal without changing the classes.
So as mentioned in the above components, this component is being used on Account and Contact record pages. Let us see how it looks on both of them.
Account Record Page:
On clicking the button, ‘See Related Contacts’, it queries the contacts under the account and displays that.
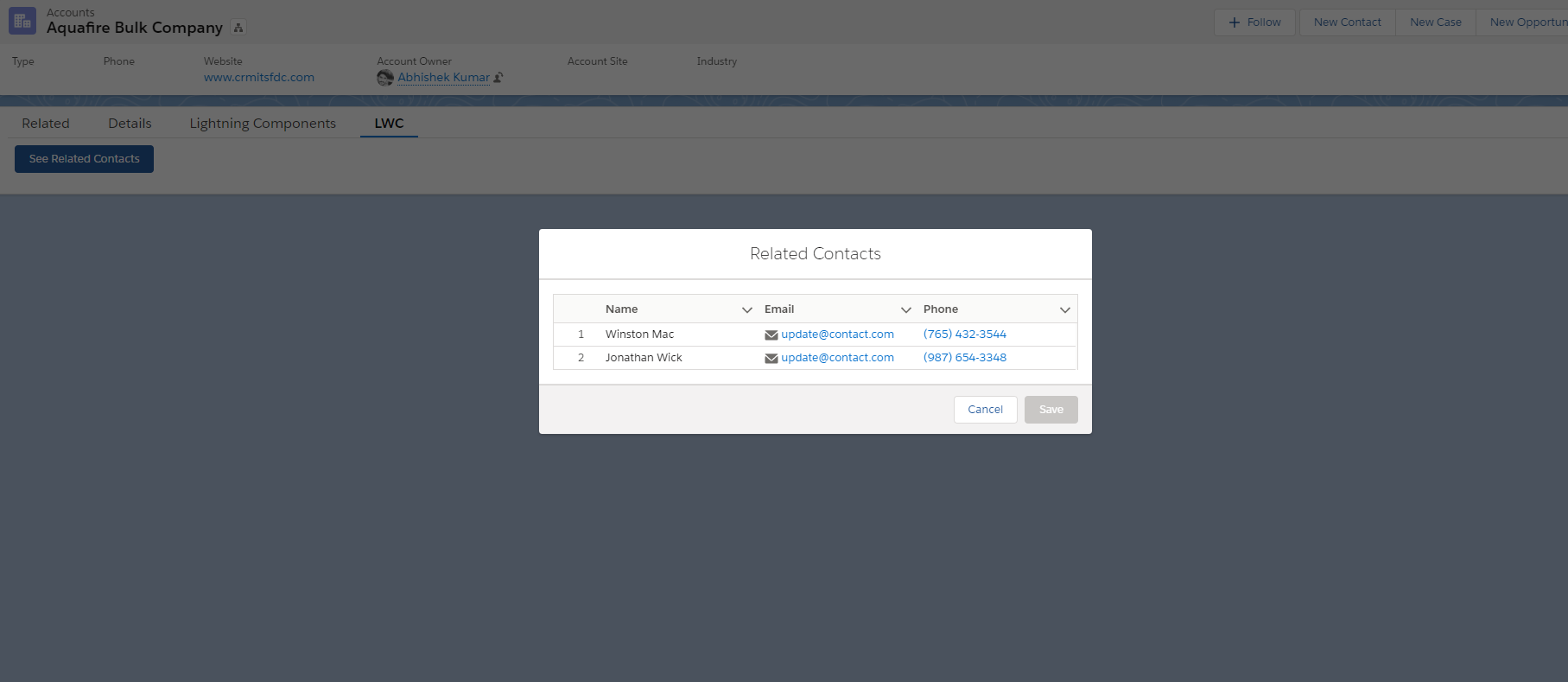
Contact Record Page:
On clicking the button, ‘See Account Details’, it fetches the related Account details and displays the fields mentioned.
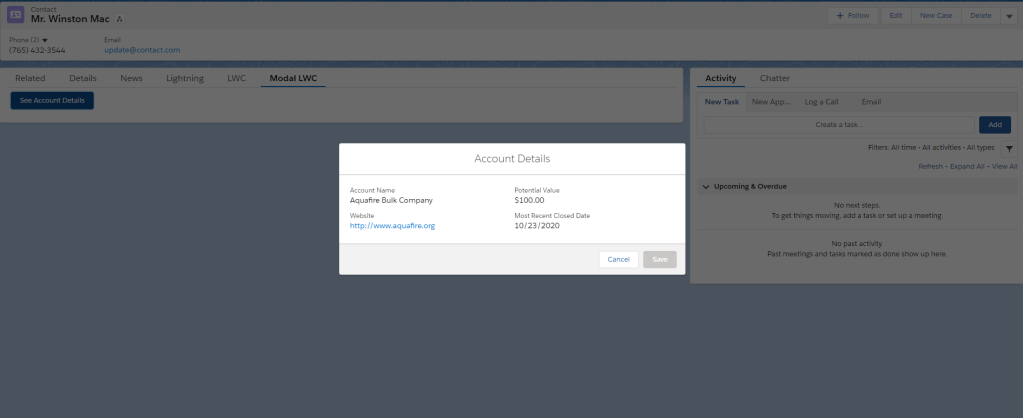
Clicking the ‘Cancel’ button, closes the Modal/ Popup.
Similarly, this component can be used to show required data in the Modal as per the sObject using this on the record page.
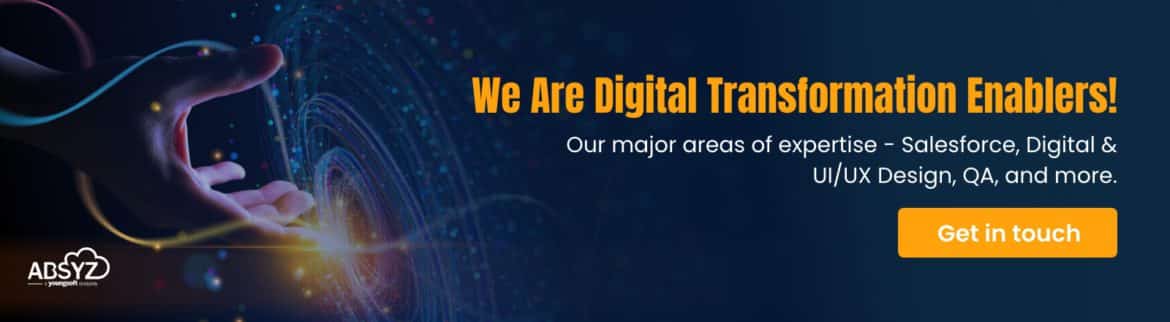